Your cart is currently empty!
Step By Step guide on integration of SendGrid in laravel
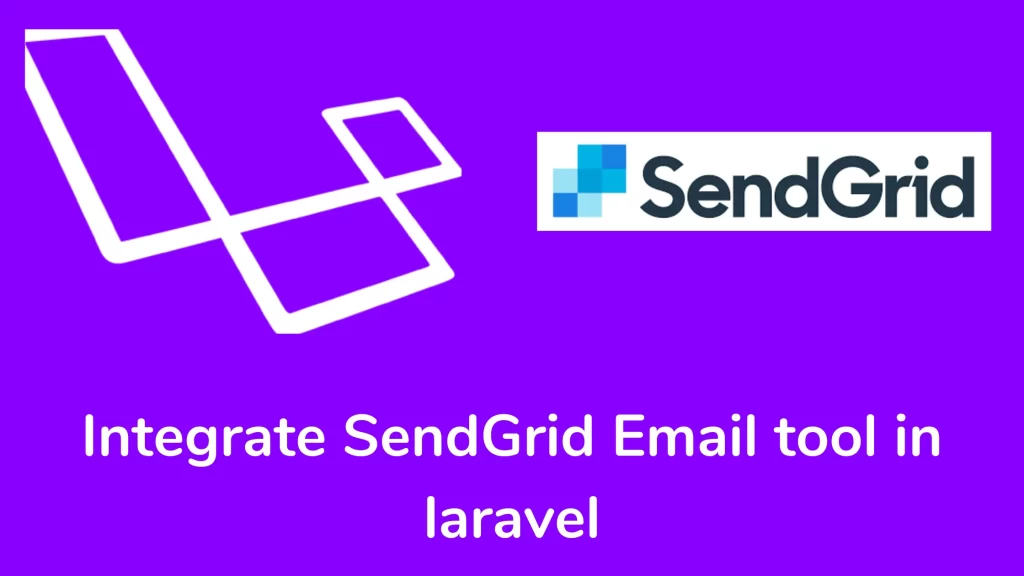
Hi guys,
SendGrid sends email in two ways:- SMTP relay and Web API. SendGrid provides client libraries in many languages. SMTP has many features by default but is hard to set up. It also provides a tracking mail API. SendGrid manages all the technical details, from scaling the infrastructure to ISP outreach and reputation monitoring to services and real-time analytics.
Step-by-step integration of SendGrid in laravel:
- Create an Account on SendGrid
If you don’t have an account on the SendGrid platform. you should create one Click Here
- Configure SendGrid credentials to an application
You can update the env file with SendGrid credentials. Look at the below code after revising the env file.
MAIL_DRIVER=smtp
MAIL_HOST=smtp.sendgrid.net
MAIL_PORT=587
MAIL_USERNAME=XXXXXXXXXXX
MAIL_PASSWORD=XXXXXXXXXXX
- Create Mail Class
You can run the below command to make a Mail class.
php artisan make:mail WelcomeMail
Above command, create a Mail class on the app/Mail directory. You can modify them according to your requirements.
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class WelcomeMail extends Mailable
{
use Queueable, SerializesModels;
public $details;
public function __construct($details)
{
$this->details = $details;
}
public function build()
{
return $this->subject('Welcome To Devrohit')
->view('emails.welcomeMail');
}
}
- Create a blade view
You can create a blade file inside the emails folder.
resources/views/emails/welcomeMail.blade.php
<html>
<head>
<title>Welcome to Devrohit
</head>
<body>
Hi {{$details['name']}},
Thank you for registering our portal. You will get the best knowledge on this platform.
{{ $deails['body']}}
<p>Thank you</p>
</body>
</html>
- Trigger mail to a recipient
you can call the send method when a user registers to your portal.
$details = [
'name'=> $user->username,
'body'=>'You are welcome to our family.'
];
\Mail::to($user->email)->send(new \App\Mail\WelcomeMail($details));
You can add the above code to the register function. Now your users will get welcome mail when they register for your application. You measure business impact on your email campaigns with a/b testing.
Now your users will get welcome mail when they register for your application. Do you want to check which mail tools are best for your application? Click Here. I hope that this post (Integrate Sendgrid Email tool in laravel) has clarified how to integrate SendGrid in laravel. If you have any questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers