Your cart is currently empty!
How to Implement login with google in laravel
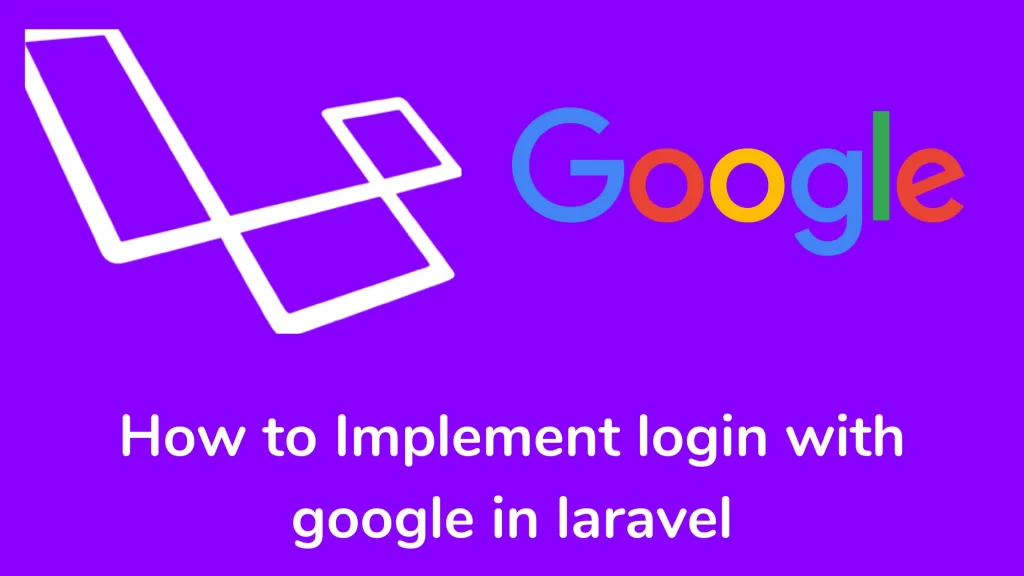
Hi guys,
Nowadays, users are less interact with the website. You want to register users on your platform. You should simplify the process of registration and login for your application. Laravel provides a socialite package to ease the process, i.e., in minimum steps, users can connect to your application. In this article, we implement login with Google in the laravel application.
Step-by-step guide on Google login in the laravel application
- Install package
Install the Socialite package on the laravel application. Run the following command.
composer require laravel/socialite
- Configure credentials
See the Screenshot for getting Google credentials. Click here to make credentials on the Google platform.


You can add credentials to the env file and add the environment variable on a config/services.php file against the Google variable like the below:
GOOGLE_AUTH_CLIENT_ID=XXXXXXXXXXXXXXXX
GOOGLE_AUTH_CLIENT_SECRET=XXXXXXXXXXXXX
GOOGLE_AUTH_URL=http://127.0.0.1:8000/auth/google
The services.php file looks like the below:
'google'=>[
'client_id' => env('GOOGLE_AUTH_CLIENT_ID'),
'client_secret' => env('GOOGLE_AUTH_CLIENT_SECRET'),
'redirect'=> env('GOOGLE_AUTH_URL')
]
- Make migration column to the user table
Make a migration file that will create a column inside the user’s table. Run migration command in your application.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddGoogleIdColumn extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function ($table) {
$table->string('google_id')->nullable();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropColumns('google_id');
}
}
Add google_id field to fillable property on User.php file.
protected $fillable = [
'name',
'email',
'password',
'google_id'
];
- Create a controller for social login
You can make a controller for social login and load the drivers for social login. I am going to load the Google driver.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Laravel\Socialite\Facades\Socialite;
use Exception;
use Inertia\Inertia;
use Illuminate\Support\Facades\Route;
use App\Models\User;
use Illuminate\Support\Facades\Auth;
use Illuminate\Support\Facades\Hash;
class SocialController extends Controller
{
public function redirectToGoogle()
{
return Socialite::driver('google')->redirect();
}
public function handleGoogleCallback()
{
try {
$user = Socialite::driver('google')->user();
$finduser = User::where('google_id', $user->id)->first();
if($finduser){
Auth::login($finduser);
return redirect('/');
}else{
$checkUser = User::where('email', $user->email)->first();
if($checkUser) {
$checkUser->google_id = $user->id;
$checkUser->save();
Auth::login($checkUser);
} else {
$newUser = User::create([
'name' => $user->name,
'email' => $user->email,
'google_id'=> $user->id,
'password' => Hash::make('123456dummy')
]);
Auth::login($newUser);
}
return redirect('/');
}
} catch (Exception $e) {
return Inertia::render('Auth/Login', [
'canResetPassword' => Route::has('password.request'),
'status' => 'Something Went wrong!! Try later',
]);
}
}
}
- Define the route
You need to define a route for social login.
Route::get('auth/google', [SocialController::class, 'redirectToGoogle']);
Route::get('auth/google/callback', [SocialController::class, 'handleGoogleCallback']);
Now we are successfully integrating Google login into your application. Socialite supports authentication via Facebook, Twitter, LinkedIn, Google, GitHub, GitLab, and Bitbucket. This service will be implemented in a couple of articles. So stay connected Or Subscribe to our newsletter. You will get notified when we publish a new article.
Now your user will easily connect to your application. Do you want to check which mail tools are best for your application? Click Here. I hope that this post (How to login with Google in laravel) has clarified how to integrate the socialite package in laravel and load the drivers. If you have any questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers
Please do not encrypt someone’s password. Hash it….
Thanks for giving a valuable comment. It is best to use the Hash algorithm for a password field.