Your cart is currently empty!
Implement Facebook login in laravel
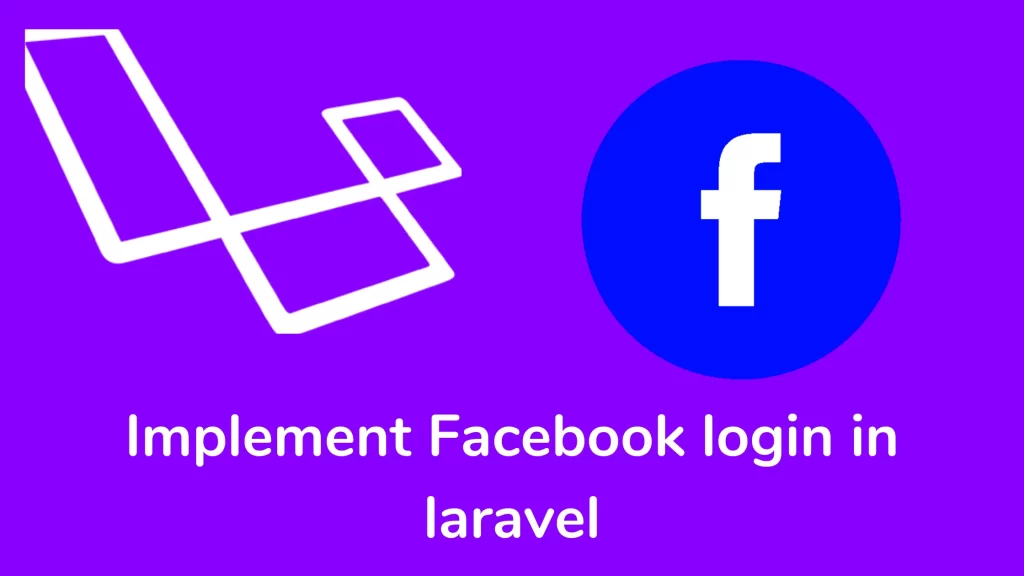
Hi guys,
Nowadays, user sessions are fewer on the website. You want to register a user on your platform. You should simplify the process of registration and login for your application. In this article, we implement a Facebook login to your application using the Socialite package.
Step-by-step guide on implementing Facebook login in Laravel
- Install the package in laravel
Run the following command to install the Socialite package in your application.
composer require laravel/socialite
You need to add the below line of code inside the config/app.php file.
$providers= [
......
Laravel\Socialite\SocialiteServiceProvider::class,
......
];
$alias = [
......
'Socialite' => Laravel\Socialite\Facades\Socialite::class,
......
]
- Configure credentials
You must create a project for getting the API Key and API Secret Key. See the below screenshots:-
You can add credentials to the env file and see the code below.
FACEBOOK_APP_ID=52097XXXXXXXXX
FACEBOOK_SECRET=59bcfb5f648d6a3cXXXXXXXXXXXX
FACEBOOK_CALLBACK_URL=http://127.0.0.1:8000/auth/facebook
You can add the below code in the services.php file.
'facebook'=>[
'client_id' => env('FACEBOOK_APP_ID'),
'client_secret' => env('FACEBOOK_SECRET'),
'redirect'=> env('FACEBOOK_CALLBACK_URL')
]
- create a column in the user table
Make a migration file that will create a column for the user’s table. Run migration command in your application.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddFacebookIdColumn extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function ($table) {
$table->string('provider');
$table->string('provider_id')->nullable();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropColumns('provider');
Schema::dropColumns('provider_id');
}
}
Run the php artisan migrate command. Add fields to fillable property on the User.php file.
protected $fillable = [
'name',
'email',
'password',
'provider',
'provider_id'
];
- Create a Controller for social login
Make a controller for social login. In your terminal, run the below command.
php artisan make:controller SocialController
We are adding two methods:
redirectToFacebook () redirects the user to Facebook to allow the site, and handleFacebookCallback () handles the user’s credentials when called back from Facebook. We load the social driver’s social login. Here, I am loading a Facebook driver.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Laravel\Socialite\Facades\Socialite;
use Exception;
use Inertia\Inertia;
use Illuminate\Support\Facades\Route;
use App\Models\User;
use Illuminate\Support\Facades\Auth;
class SocialController extends Controller
{
public function redirectToFacebook()
{
return Socialite::driver('facebook')->redirect();
}
public function handleFacebookCallback()
{
try {
$userInfo = Socialite::driver('facebook')->user();
$finduser = User::where('provider_id', $user->id)->first();
if($finduser){
Auth::login($finduser);
return redirect('/');
}else{
$checkUser = User::where('email', $user->email)->first();
if($checkUser) {
$checkUser->provider = 'facebook';
$checkUser->provider_id = $userInfo->id;
$checkUser->save();
Auth::login($checkUser);
} else {
$newUser = User::create([
'name' => $userInfo->name,
'email' => $userInfo->email,
'provider'=> 'facebook',
'provider_id'=> $userInfo->id,
'password' => encrypt('123456dummy')
]);
Auth::login($newUser);
}
return redirect('/');
}
} catch (Exception $e) {
return Inertia::render('Auth/Login', [
'canResetPassword' => Route::has('password.request'),
'status' => 'Something Went wrong!! Try later',
]);
}
}
}
- Define the route
You need to define a route for social login in the route/web.php file.
Route::get('auth/facebook', [SocialController::class, 'redirectToFacebook']);
Route::get('auth/facebook/callback', [SocialController::class, 'handleFacebookCallback']);
- Updating the login view
Open resources/views/auth/login.blade.php. Add the below code to your view.
<div class="form-group">
<div class="col-md-8 col-md-offset-4">
<a href="{{url('/auth/facebook')}}" class="btn btn-secondary">Login with Facebook</a>
</div>
</div>
Finally Done ✔️
Now we have successfully implemented Facebook login into your application. Go to the application login page and click on the login with Facebook button. It redirected the user to the GitHub authorization page. The user authorizes the application, and it will redirect to the dashboard page.
Do you want to check which mail tools are best for your application? Click Here. If you have any questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers