Your cart is currently empty!
How to Create Custom Facade in laravel?
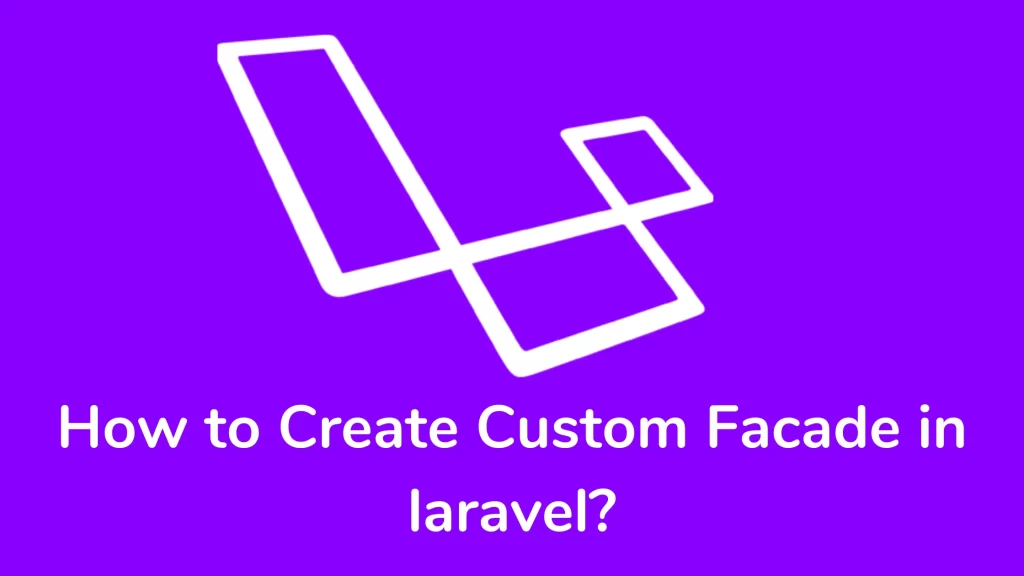
Hi Guys,
In this article, We are studying facades in laravel. A facade is a design pattern that provides a static interface to classes inside the framework’s service container. You can access all the features of laravel with facades. It provides the benefit of a terse, expressive syntax while maintaining more testability and flexibility.
Some of the laravel facades
- Cache: Access the caching system.
- Config: Access the configuration values.
- DB: Interact with the database using Laravel’s query builder
- Log: write a log message
- Mail: Access the Mailing system.
- File: Perform file operation
- Route: handle HTTP request
- Storage: handle file system.
- Session: Manage session data
Laravel contains many facades to handle the core functionality of laravel. Also, you can create custom facades that allow you to access them more concisely throughout your application.
How to create a custom Facade in Laravel
Create a helper class
Create a “Message” directory inside the App directory and create a php class inside the directory.
<?php
namespace App\Message;
class WelcomeMessage
{
public function greet()
{
return 'Welcome to Our Platform';
}
}
Register helper class
You can register a helper class in AppServiceProvider Provider.
public function register()
{
$this->app->bind('greeting', function(){
return new WelcomeMessage();
});
}
Create a Facade class
create a WelcomeFacade class in the “Message” directory, which extends Illuminate\Support\Facades\Facade.
<?php
namespace App\Message;
use Illuminate\Support\Facades\Facade;
class WelcomeFacade extends Facade
{
protected static function getFacadeAccessor()
{
return 'greeting';
}
}
Register the Facade in the config file
You can add an entry in alias in config/app.php
<?php
return [
'alias' => [
'Welcome' => App\Message\WelcomeFacade::class,
],
];
?>
Implement on view page
We can use this Facade to showcase the message return from Facades.
Route::get('/welcome', function(){
return response(Welcome::greet(), 200);
});
You can use this in any place. So you can display messages.
We have implemented custom facades in your application. It provides a static interface to dynamic methods of underlying classes, allowing for clean and expressive syntax in code. Facades simplify the usage of complex components, making them accessible via familiar static methods, and enhancing the readability and maintainability of Laravel applications.
You can check developer tools on the Supertools website. Please give me feedback on this website.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers