Your cart is currently empty!
How to Implement Contracts in laravel application
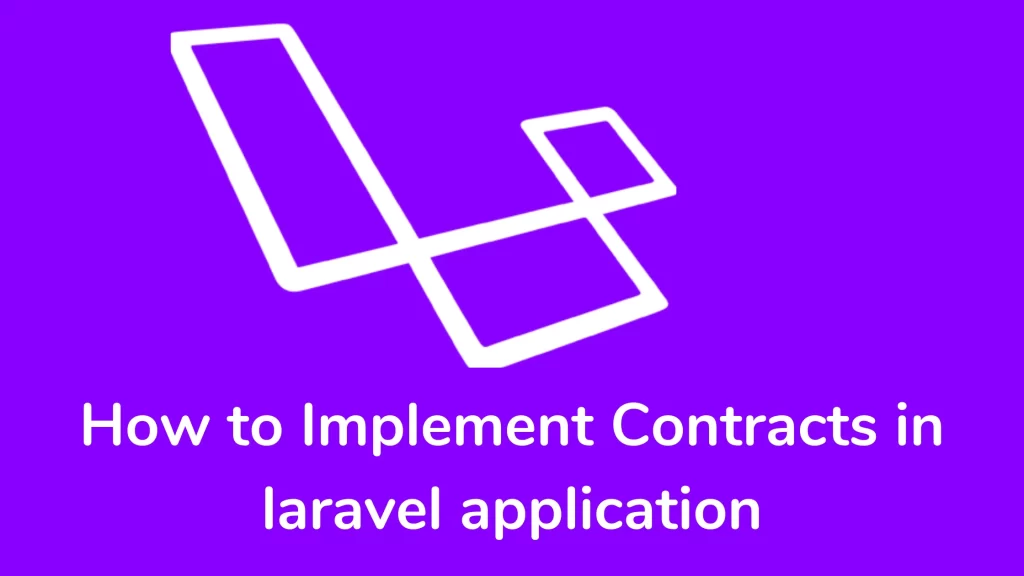
Hi,
Laravel contracts are a set of interfaces that define the core services. It provides a queue implementation with a variety of drivers. Laravel provides you with a quick reference point for all available contracts.
Contracts v/s Facades:-
Contracts need to have explicit dependencies on your classes. In general, most applications can use facades without issue during development. The Facades do not require you to require them in your class constructor.
How to Implement Contracts in Laravel Application
For implementing a contract, you can just “type-hint” the interface in the class’s constructor. Check the code given below:
<?php
namespace App\Listeners;
use App\Events\OrderWasPlaced;
use App\Models\User;
use Illuminate\Contracts\Redis\Factory;
class CacheOrderInformation
{
/**
* Create a new event handler instance.
*/
public function __construct(
protected Factory $redis,
) {}
/**
* Handle the event.
*/
public function handle(OrderWasPlaced $event): void
{
// ...
}
}
Contract Reference:-
Below is the list of contracts and facades, which are equivalent to each other. You can use it according to your requirements.
The decision to use contracts or facades will come down to personal taste and the tastes of your development team. I am using Facades inside the laravel project. All the Laravel Contracts live in their own GitHub repository. It provides a quick reference point for all available contracts.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers