Your cart is currently empty!
How to implement bitbucket login in laravel
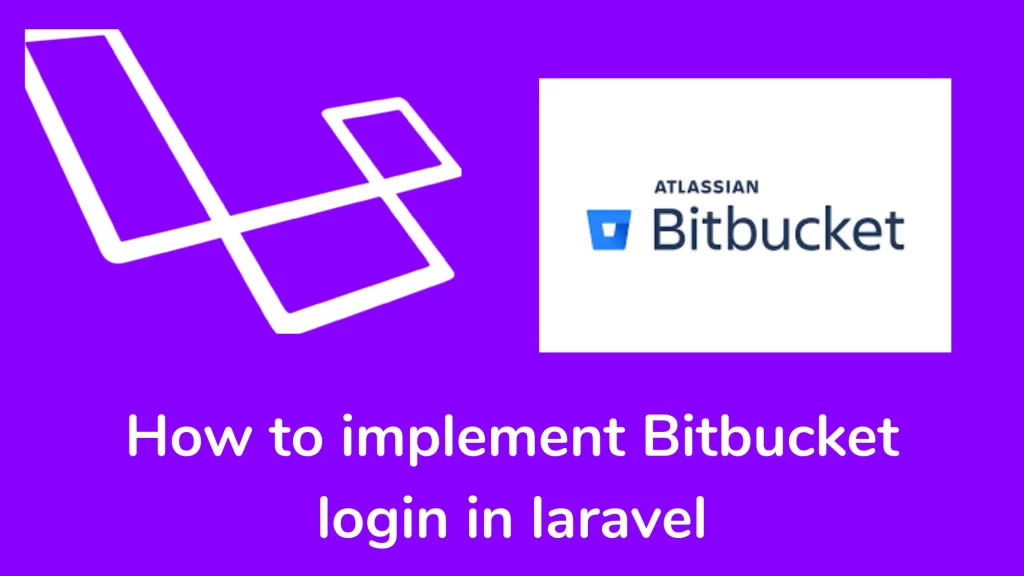
Hi guys,
According to a research report, users’ sessions are shorter on every website nowadays. In this case, you can simplify the registration and login process for your application. The Socialite package is the best and primarily used package for social login. It is used to reduce the process. In this article, we implement a Bitbucket login to your application. Are you looking for a Google or Twitter login? you can visit our social login series for your knowledge.
Step-by-step guide on Bitbucket login in laravel application
- Install Socialite Package
Install the Socialite package on the laravel application. Run the following command.
composer require laravel/socialite
You need to add the below line of code inside the app.php file.
$providers= [
......
Laravel\Socialite\SocialiteServiceProvider::class,
......
];
$alias = [
......
'Socialite' => Laravel\Socialite\Facades\Socialite::class,
......
]
- Configure credentials
Go to the Bitbucket page to get App credentials for your application.
You can add credentials to the env file and add the environment variable on services.php against the Bitbucket variable.
BITBUCKET_APP_ID=XXXXXXXXXX
BITBUCKET_SECRET=XXXXXXXX
BITBUCKET_CALLBACK_URL=http://127.0.0.1:8000/auth/bitbucket
Add the below code in the services.php file.
'bitbucket'=>[
'client_id' => env('BITBUCKET_APP_ID'),
'client_secret' => env('BITBUCKET_SECRET'),
'redirect'=> env('BITBUCKET_CALLBACK_URL')
]
- create a column in the user table
Make a migration file that will create a column for the users’ table. Run migration command in your application.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddBitbucketIdColumn extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function ($table) {
$table->string('provider');
$table->string('provider_id')->nullable();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropColumns('provider');
Schema::dropColumns('provider_id');
}
}
Run the php artisan migrate command. Add fields to fillable property on the User.php file.
protected $fillable = [
'name',
'email',
'password',
'provider',
'provider_id'
];
- Create a Controller for social login
Make a controller for social login. In your terminal, run the following command.:
php artisan make:controller SocialController
We are adding two methods: redirectToBitbucket(), which redirects the user to Bitbucket to authorize the site, and handleBitbucketCallback(), which handles the user’s credentials when called back from Bitbucket. We load the social driver’s social login. Here, I am loading a bitbucket driver.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Laravel\Socialite\Facades\Socialite;
use Exception;
use Inertia\Inertia;
use Illuminate\Support\Facades\Route;
use App\Models\User;
use Illuminate\Support\Facades\Auth;
class SocialController extends Controller
{
public function redirectToBitbucket()
{
return Socialite::driver('bitbucket')->redirect();
}
public function handleBitbucketCallback()
{
try {
$userInfo = Socialite::driver('bitbucket')->user();
$finduser = User::where('provider_id', $user->id)->first();
if($finduser){
Auth::login($finduser);
return redirect('/');
}else{
$checkUser = User::where('email', $user->email)->first();
if($checkUser) {
$checkUser->provider = 'bitbucket';
$checkUser->provider_id = $userInfo->id;
$checkUser->save();
Auth::login($checkUser);
} else {
$newUser = User::create([
'name' => $userInfo->name,
'email' => $userInfo->email,
'provider'=> 'bitbucket',
'provider_id'=> $userInfo->id,
'password' => encrypt('123456dummy')
]);
Auth::login($newUser);
}
return redirect('/');
}
} catch (Exception $e) {
return Inertia::render('Auth/Login', [
'canResetPassword' => Route::has('password.request'),
'status' => 'Something Went wrong!! Try later',
]);
}
}
}
- Define the route
You need to define a route for social login in the route/web.php file.
Route::get('auth/bitbucket', [SocialController::class, 'redirectToBitbucket']);
Route::get('auth/bitbucket/callback', [SocialController::class, 'handleBitbucketCallback']);
- Updating the login view
Open resources/views/auth/login.blade.php. Add the below code and change the button style according to your application interface.
<div class="form-group">
<div class="col-md-8 col-md-offset-4">
<a href="{{url('/auth/bitbucket')}}" class="btn btn-secondary">Login with Bitbucket</a>
</div>
</div>
Finally Done ✔️
Now we have successfully integrated Bitbucket login into your application. Go to the application login page and click on the login with the Bitbucket button. It redirected the user to the Bitbucket authorization page. After authorization, it will redirect the user to your application.
Now your user will easily connect to your application. Do you want to check which mail tools are best for your application? Click Here. I hope that this post (How to implement Bitbucket login in laravel) has clarified how to integrate the Socialite package in laravel and load the drivers. If you have questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers